Spring IoC & Dependency Injection: A Beginner’s Guide with Diagrams
This blog documents my learning journey about Spring' Inversion of Control and Dependency Injection. I hope this helps you as much as it is helping for me. In this blog, I'll walk you through:
- Spring IoC and Dependency Injection explained visually
- UML Class Diagrams to model DI relationships
- Sequence Diagrams to show how Spring initializes beans
- Maven Project Setup for building and packaging a Spring IoC application
Let's create an example where we have a Car
class that depends on an Engine
class. We'll use Spring IoC and Dependency Injection (DI) using Spring's ApplicationContext. ApplicationContext is an IoC container that manages beans. The Car
class does not create an Engine object itself. Instead, it gets the Engine object from the ApplicationContext. This is called Dependency Injection (DI).
Here is the UML Class Diagram for the Car and Engine classes and sequence diagram to show the flow of dependency injection in Spring:
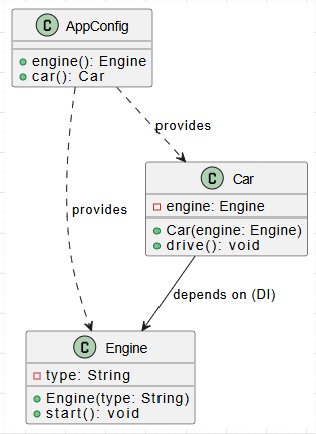
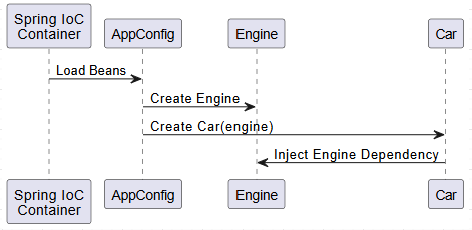
Let's create a Maven project with the following structure:
.
├── README.md
├── pom.xml
└── src
├── main
│ ├── java
│ │ └── org
│ │ └── example
│ │ ├── AppConfig.java (Spring configuration)
│ │ ├── Car.java (depends on Engine)
│ │ ├── Engine.java (dependency)
│ │ └── Main.java
│ └── resources
└── test
└── java
Let's define the Engine
class.
public class Engine {
private String type;
public Engine(String type) {
this.type = type;
}
public String getType() {
return type;
}
}
public class Car {
private Engine engine;
// Constructor-based Dependency Injection
public Car(Engine engine) {
this.engine = engine;
}
public void drive() {
System.out.println("Car is driving with " + engine.getType() + " engine");
}
}
We configure Spring with Java-based configuration.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public Engine engine() {
return new Engine("V8");
}
@Bean
public Car car() {
return new Car(engine()); // Injecting Engine bean
}
}
Let's define Main
class to run the Spring IoC container.
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
// Load Spring IoC container using Java-based configuration
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
// Get the Car bean
Car myCar = context.getBean(Car.class);
// Use the Car object
myCar.drive();
}
}
You can clone the project from my GitHub repository: GitHub Repository